What are environment variables
Environment variables are dynamic values that can be used by applications to configure themselves without hardcoding sensitive or environment-specific information directly into the code. They are typically used to store settings like API keys, database connection strings, or other configuration details that change between development, staging, and production environments.
How and where to create .env file
Environment variables are stored inside so called .env files. You can add any suffix you'd like, but the ".env" part has to be present. For your apps a recommended approach would be that for each environment you would have separate .env file. So you would have:
- Local -> .env.local
- Development -> .env.development
- Staging -> .env.staging
- Production -> .env.production
Now the question where do you create your .env files? You should always have those files created in the root of your project. For an example: In React app you would have the .env files on the same level as src folder. In NextJs app you would have the .env files on the same level as app folder.
.env configuration
After you create a .env file you need to know what to put inside these files. So the naming of these can sometimes be tricky, but here below you can see a basic name value pairs. You have a name that should be in all caps separated with _ , then you have to have the equal sign (=) and then the actual value you need to save. This value can be anything you want. This value will always be a string type. You have to keep in mind that you will have to cast your values according to your use-case.
1DB_PASSWORD=ASAgency123
2DB_NAME=example-db
Accessing your .env values
This can also be a tricky one. The first step for you would be to check what module bundler you are using. Let's say you're using Vite. This next code snippet show how you access vite .env variable
1const openAPIKey = import.meta.env.VITE_APP_OPEN_API_KEY
So for Vite we use import.meta.env.
Let's say now you're using NextJs or vanilla JS for your project. These don't use Vite so you have to access your .env values in a different way.
1const openAPIKey = process.env
These are two ways of importing your .env values. If you're using Vite you use the first approach and if you're not using it you use the second approach.
Adding types
As a first step lets create a .env file in root of your project.
1VITE_APP_OPEN_API_KEY=this-is-my-key
When you try to access your .env values, you wont get any type suggestions.
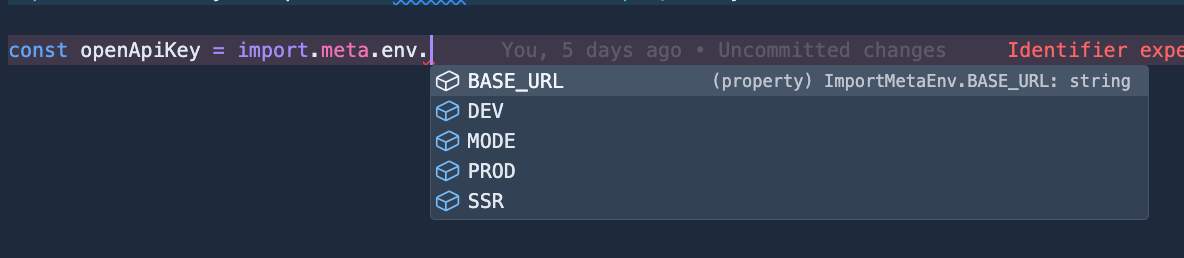
Slika: Type suggestions
As you can see there is no open api key in the suggestions. How do we fix this?
The solutions is easier than you think!
First step is to create a declaration file (d.ts) where you will add and configure your .env types. This can be created anywhere but best practice would be that you create a @types folder in root of your project and then inside that folder you create enviroment.d.ts file (can be named anything.)
In this file for your Vite configuration you will need:
1/// <reference types="vite/client" />
2
3interface ImportMetaEnv {
4 readonly VITE_APP_OPEN_API_KEY: string;
5}
6
7interface ImportMeta {
8 readonly env: ImportMetaEnv;
9}
10
And then in ImportMetaEnv add your environment variables, this then extends the vite env type with your types.
And for non vite projects you will need the next configuration:
1declare namespace NodeJs {
2 export interface ProcessEnv {
3 OPEN_API_KEY: string;
4 }
5}
And the final result should look like:
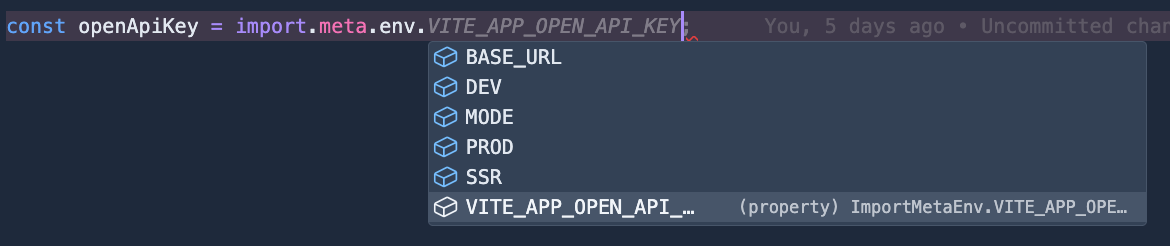
Slika: Type suggestion
As you can see, now we have our variable name as a type suggestion.
To learn more or to work with us, contact us trough the form on this link:
Contact us
Or send a message or call directly on: +385 97 652 2509

Slika: